C#面向对象实现图书管理系统
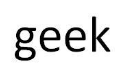
这篇文章主要为大家详细介绍了C#面向对象实现图书管理系统,文中示例代码介绍的非常详细,具有一定的参考价值,感兴趣的小伙伴们可以参考一下
本文实例为大家分享了C#面向对象实现图书管理系统的具体代码,供大家参考,具体内容如下
这个系统需要两个类:class Book,class BookManager
首先先进行对Book类成员的声明。
class Book { //数据 string id; public string Id { get { return id; } set { id = value;} } //使用了lamda表达式, 跟上面的get/set等价 public string Name { get => name; set => name = value; } string name; string author; float price; int page; public int Page { get; set; } public void print() { string str = string.Format("书名是{0},作者是{1},编号是{2},价格{3},页数{4}", name,author,id,price,page); Console.WriteLine(str); } }
BookManager类的声明
//数据成员 int size;//容器的大小 public int curIndex;//当前存储的位置 Book[] books; //定义一个Book类型的数组 //函数成员 public BookManager(int size) { curIndex = 0; this.size = size; //申请了一个容器,目前里面还没有值 books = new Book[size]; }
实现添加的方法
1、curIndex一开始的位置是[0],将b的内存地址存入数组中的第0位后,curIndex+1,这样的话下一次存储时会将数据存储到第1位。
2、判断数组的游标是否大于容器大小, 大于则要进行数组扩容
public void addBook(Book b)//传入Book的一个引用 b { books[curIndex] = b;//将b存入数组 curIndex++; if (curIndex>=size)//判断数组的游标是否大于容器大小, 大于则要进行数组扩容 { size = size + size / 2;//将容器的大小扩容增加原来的一半 Book[] booksNew = new Book[size];//定义一个新的Book类型数组 booksNew Array.Copy(books,booksNew, books.Length);//使用Copy函数将books数组里面的值赋值给booksNew,长度为books的长度 books = booksNew; } }
重写删除的文本,通过ID遍历
public bool delBook(string id) { int findCurIndex = 0; bool isFind = false; for (int i = 0; i < curIndex; i++)//通过遍历比较两个id { if (books[i].Id.Equals(id)) { findCurIndex = i; isFind = true; break; } } return false; }
查找书籍方法
public Book find(string id) { if (string.IsNullOrEmpty(id)) { return null;//返回空引用 } for (int i = 0; i < curIndex; i++)//通过遍历比较两个id { if (books[i]!=null&&books[i].Id.Equals(id)) { return books[i]; } } return null; }
显示所有书籍方法
public void showAllBook() { Console.WriteLine("所有的书籍信息如下"); for (int i = 0; i < curIndex; i++) { books[i].print(); } }
以上,书和管理器的类就写完了 接下来在主函数中运行测试:
using System; namespace 图书管理系统 { class Program { static void Main(string[] args) { Console.WriteLine("欢迎进入图书管理软件"); int num = 1; BookManager manager = new BookManager(2);//容器(数据库) while (true) { Console.Clear();//清理控制台信息 Console.WriteLine("1、录入书籍\n2、查找书籍\n3、删除书籍\n4、显示所有书籍\n按对应的数字进入该功能,按Q退出"); string str = Console.ReadLine(); num = int.Parse(str); switch (num) { case 1://录入书籍 Console.WriteLine("已经进入录入书籍功能"); Console.Write("输入编号:"); string id = Console.ReadLine(); Console.Write("输入书名:"); string name = Console.ReadLine(); Console.Write("输入作者名字:"); string authName = Console.ReadLine(); Console.Write("输入书的价格:"); float price = float.Parse(Console.ReadLine()); Console.Write("输入书的页数:"); int page = int.Parse(Console.ReadLine()); Book book = new Book(authName, price);//通过构造函数生成对象,并且赋值 //通过属性对对象赋值 book.Id = id; book.Name = name; book.Page = page; //把书存储到管理器中 manager.addBook(book); break; case 2://查找书籍 if (manager.curIndex == 0) { Console.Write("系统里一本书都没有!"); break; } id = Console.ReadLine(); Book curBook = manager.find(id); if (curBook != null) { Console.WriteLine("找到了书,信息如下:"); curBook.print(); } else { Console.WriteLine("没有找到书"); } break; case 3://删除书籍 Console.Write("输入要删除的书籍编号:"); id = Console.ReadLine(); Book _curBook = manager.find(id); if (_curBook != null) { Console.WriteLine("已删除{0}这本书", _curBook.Name); manager.delBook(_curBook.Id); } else { Console.WriteLine("没有找到书"); } break; case 4://显示所有书籍 if (manager.curIndex != 0) { manager.showAllBook(); } else { Console.WriteLine("系统里一本书都没有!"); } break; } if (str.ToLower().Contains('q')) { break; } Console.ReadKey(); } } } }
相关推荐
PHP实现部分字符隐藏
沙雕mars · 1325浏览 · 2019-04-28 09:47:56

Java中ArrayList和LinkedList区别
kenrry1992 · 908浏览 · 2019-05-08 21:14:54

5月语言排行榜:R 跌出前二十,Python 紧咬 C++
manongba · 687浏览 · 2019-05-09 17:27:24

Tomcat 下载及安装配置
manongba · 970浏览 · 2019-05-13 21:03:56

什么是SpringBoot
iamitnan · 1086浏览 · 2019-05-14 22:20:36

分类专栏
最新发布
最热排行
0评论